In this tutorial you will learn about the Laravel 8 Rest API CRUD with Passport Auth Tutorial and its application with practical example.
In this Laravel 8 Rest API CRUD with Passport Auth Tutorial tutorial, I will show you how to create rest api in laravel 8 application with passport authentication. In this tutorial we will be using passport for api authentication. we will create register and login api with simple retrieve user details.
Laravel 8 Rest CRUD APIs Tutorial With Example
- Download Laravel 8 App
- Database Configuration
- Install Passport Auth
- Passport Configuration
- Create Product Table and Model
- Run Migration
- Create Auth and CRUD APIs Route
- Create Passport Auth and CRUD Controller
- Test Laravel 8 REST CRUD API with Passport Auth in Postman
Install Laravel 8
First of all we need to create a fresh laravel project, download and install Laravel 8 using the below command
1 |
composer create-project --prefer-dist laravel/laravel larablog |
Configure Database In .env file
Now, lets create a MySQL database and connect it with laravel application. After creating database we need to set database credential in application’s .env file.
1 2 3 4 5 6 |
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=larablog DB_USERNAME=root DB_PASSWORD= |
Install Passport Package
In this step, we need to install Laravel Passport package via the composer dependency manager. Use the following command to install passport package.
1 |
composer require laravel/passport |
After Installing ‘laravel/passport’ package, we need to add service provider in config/app.php file as following.
config/app.php
1 2 3 4 |
'providers' => [ .... Laravel\Passport\PassportServiceProvider::class, ] |
Run Migration and Install Laravel Passport
After successfully installing ‘laravel/passport’ package, we require to create default passport tables in our database. so let’s run the following command to migrate Laravel Passport tables to your database.
1 |
php artisan migrate |
Now, it is mandatory to install passport using the command below. This command will generate encryption keys required to generate secret access tokens.
1 |
php artisan passport:install |
Laravel Passport Configuration
Now, we need to make following changes in our model, service provider and auth config file to complete passport configuration. Open App/User.php model file and add ‘Laravel\Passport\HasApiTokens’ trait in it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
<?php namespace App\Models; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Laravel\Passport\HasApiTokens; class User extends Authenticatable { use Notifiable, HasApiTokens; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast to native types. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; } |
Next Register passport routes in App/Providers/AuthServiceProvider.php, open App/Providers/AuthServiceProvider.php and put “Passport::routes()” inside the boot method like below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
<?php namespace App\Providers; use Laravel\Passport\Passport; use Illuminate\Support\Facades\Gate; use Illuminate\Foundation\Support\Providers\AuthServiceProvider as ServiceProvider; class AuthServiceProvider extends ServiceProvider { /** * The policy mappings for the application. * * @var array */ protected $policies = [ 'App\Model' => 'App\Policies\ModelPolicy', ]; /** * Register any authentication / authorization services. * * @return void */ public function boot() { $this->registerPolicies(); } } |
Now open config/auth.php file and set api driver to passport instead of session.
1 2 3 4 5 6 7 8 9 10 |
[ 'web' => [ 'driver' => 'session', 'provider' => 'users', ], 'api' => [ 'driver' => 'passport', 'provider' => 'users', ], ], |
Create Product Table and Model
Now we will create product model and migration file, open terminal and run the following command:
1 |
php artisan make:model Product -m |
After that, navigate to database/migrations directory and open create_products_table.php file. Then put the following code in it:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateProductsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('products', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('name'); $table->text('detail'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('products'); } } |
Now, add fillable property in product.php file, so go to app/models directory and open product.php file and put the following code into it:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'detail' ]; } |
Run Migration
Now you need to run the migration using the following command. This command will create tables in the database :
1 |
php artisan migrate |
Create Auth and CRUD APIs Route
Now we will create rest API auth and crud operation routes. Go to the routes directory and open api.php. Then put the following routes into api.php file:
routes/api.php
1 2 3 4 5 6 7 8 9 10 11 12 |
use App\Http\Controllers\API\PassportAuthController; use App\Http\Controllers\API\ProductController; Route::post('register', [PassportAuthController::class, 'register']); Route::post('login', [PassportAuthController::class, 'login']); Route::middleware('auth:api')->group(function () { Route::get('get-user', [PassportAuthController::class, 'userInfo']); Route::resource('products', [ProductController::class]); }); |
Create Passport Auth and CRUD Controller
In this step, we will create a controllers name PassportAuthController and ProductController. Use the following command to create a controller :
1 2 |
php artisan make:controller Api\PassportAuthController php artisan make:controller Api\ProductController |
After that, Create some authentication methods in PassportAuthController.php. So navigate to app/http/controllers/API directory and open PassportAuthController.php file. And, update the following methods into your PassportAuthController.php file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
<?php namespace App\Http\Controllers\API; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use App\Models\User; class AuthController extends Controller { /** * Registration Req */ public function register(Request $request) { $this->validate($request, [ 'name' => 'required|min:4', 'email' => 'required|email', 'password' => 'required|min:8', ]); $user = User::create([ 'name' => $request->name, 'email' => $request->email, 'password' => bcrypt($request->password) ]); $token = $user->createToken('Laravel8PassportAuth')->accessToken; return response()->json(['token' => $token], 200); } /** * Login Req */ public function login(Request $request) { $data = [ 'email' => $request->email, 'password' => $request->password ]; if (auth()->attempt($data)) { $token = auth()->user()->createToken('Laravel8PassportAuth')->accessToken; return response()->json(['token' => $token], 200); } else { return response()->json(['error' => 'Unauthorised'], 401); } } public function userInfo() { $user = auth()->user(); return response()->json(['user' => $user], 200); } } |
Now we will create rest api crud methods in ProductController.php. So navigate to app/http/controllers/API directory and open ProductController.php file. And, update the following methods into your ProductController.php file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
<?php namespace App\Http\Controllers\API; use App\Http\Controllers\Controller; use App\Models\Product; use Illuminate\Http\Request; use Validator; class ProductController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $products = Product::all(); return response()->json([ "success" => true, "message" => "Product List", "data" => $products ]); } /** * Store a newly created resource in storage. * * @param \Illuminate\Http\Request $request * @return \Illuminate\Http\Response */ public function store(Request $request) { $input = $request->all(); $validator = Validator::make($input, [ 'name' => 'required', 'detail' => 'required' ]); if($validator->fails()){ return $this->sendError('Validation Error.', $validator->errors()); } $product = Product::create($input); return response()->json([ "success" => true, "message" => "Product created successfully.", "data" => $product ]); } /** * Display the specified resource. * * @param int $id * @return \Illuminate\Http\Response */ public function show($id) { $product = Product::find($id); if (is_null($product)) { return $this->sendError('Product not found.'); } return response()->json([ "success" => true, "message" => "Product retrieved successfully.", "data" => $product ]); } /** * Update the specified resource in storage. * * @param \Illuminate\Http\Request $request * @param int $id * @return \Illuminate\Http\Response */ public function update(Request $request, Product $product) { $input = $request->all(); $validator = Validator::make($input, [ 'name' => 'required', 'detail' => 'required' ]); if($validator->fails()){ return $this->sendError('Validation Error.', $validator->errors()); } $product->name = $input['name']; $product->detail = $input['detail']; $product->save(); return response()->json([ "success" => true, "message" => "Product updated successfully.", "data" => $product ]); } /** * Remove the specified resource from storage. * * @param int $id * @return \Illuminate\Http\Response */ public function destroy(Product $product) { $product->delete(); return response()->json([ "success" => true, "message" => "Product deleted successfully.", "data" => $product ]); } } |
Then open command prompt and run the following command to start developement server:
1 |
php artisan serve |
Test Laravel 8 REST CRUD API with Passport Auth in Postman
Now, we will call above create crud and auth apis in postman app:
1 – Laravel Register Rest API :
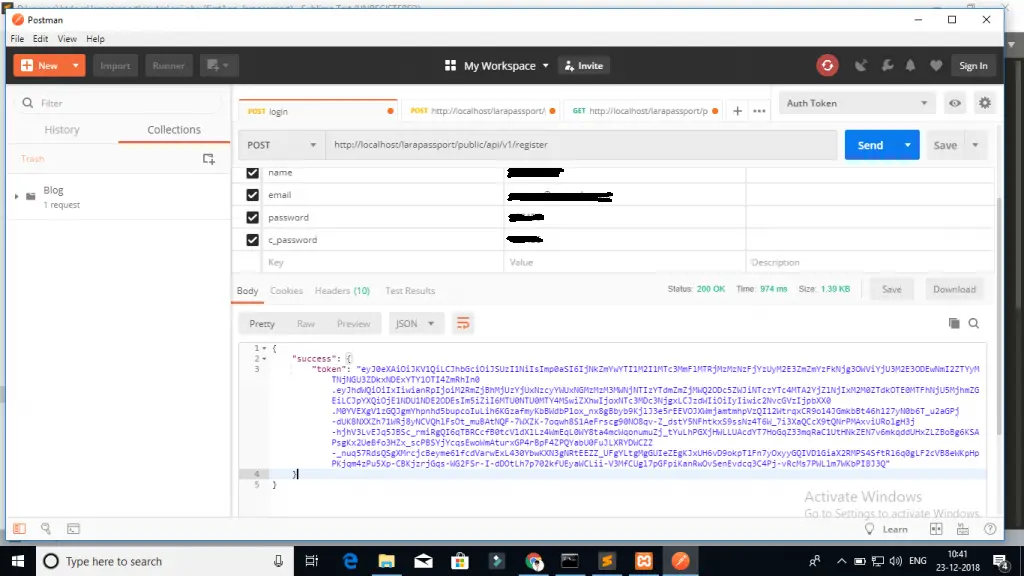
2 – Login API :
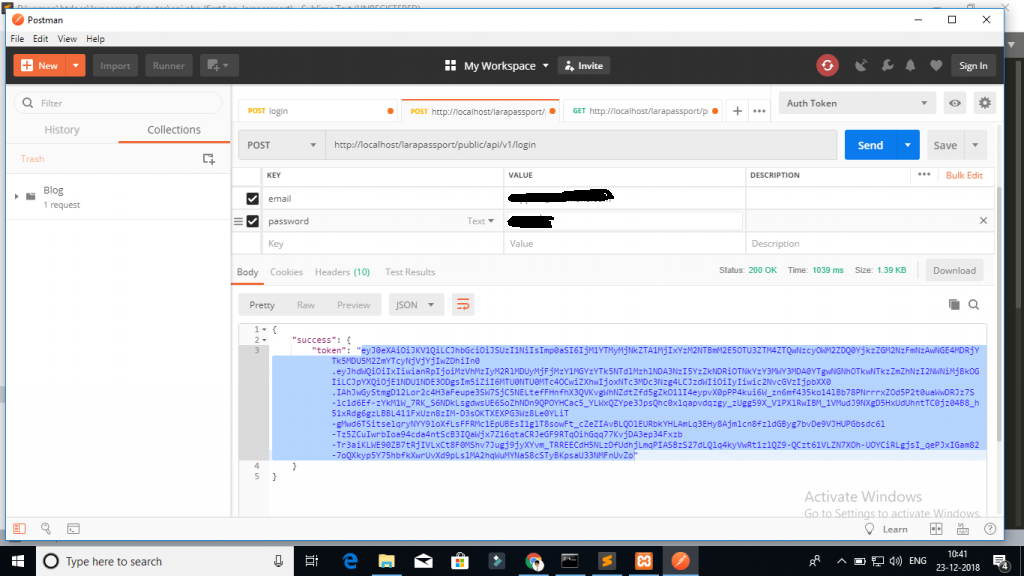
Next Step, you will call getUser, create product, list product, edit product, and delete product APIs, In this apis need to pass the access token as headers:
1 2 3 4 |
‘headers’ => [ ‘Accept’ => ‘application/json’, ‘Authorization’ => ‘Bearer ‘.$accessToken, ] |
3 – Product List API
- Method:- GET
- URL:- http://127.0.0.1:8000/api/products
4 – Product Create API
- Method:- POST
- URL:- http://127.0.0.1:8000/api/products
5 – Product Fetch API
- Method:- GET
- URL :- http://127.0.0.1:8000/api/products/{id}
6 – Product Update API
- Method:- PUT
- URL :- http://127.0.0.1:8000/api/products/{id}
7 – Product Delete API
- Method:- DELETE
- URL :- http://127.0.0.1:8000/api/products/{id}